

Certainly! Let’s enhance the menu by adding descriptions for each option to explain what each function does. This way, users will have a clear understanding of each action.
“`bash
#!/bin/bash
# Function to define project goals
defineProjectGoals() {
echo “Function: Define Project Goals”
echo “Actions:”
echo “- Set clear financial and community engagement goals.”
echo “- Define milestones for both financial and community progress.”
echo “- Establish a timeline for achieving project goals.”
}
# Command to assemble the team
assembleTeam() {
echo “Function: Assemble Team”
echo “Actions:”
echo “- Clone the project repository.”
echo “- Create and switch to a new feature branch.”
echo “- Add and commit initial setup changes.”
}
# Command to encourage open source
encourageOpenSource() {
echo “Function: Encourage Open Source”
echo “Actions:”
echo “- Fork the project to encourage open-source contributions.”
echo “- Create a pull request for collaboration.”
echo “- Create an enhancement issue to suggest improvements.”
}
# Command to implement governance model
implementGovernanceModel() {
echo “Function: Implement Governance Model”
echo “Actions:”
echo “- Create a decentralized autonomous organization (DAO).”
echo “- Install a voting app for community decision-making.”
echo “- Vote on a proposal using the AragonCLI.”
}
# Command to implement emotional features
implementEmotionalFeatures() {
echo “Function: Implement Emotional Features”
echo “Actions:”
echo “- Use slack-term to implement emotional features in Slack.”
echo “- Send positive messages to create a happy atmosphere.”
echo “- Share happy images using slack-term.”
}
# Command to gather user feedback
gatherUserFeedback() {
echo “Function: Gather User Feedback”
echo “Actions:”
echo “- Use curl to make a GET request to the survey API.”
echo “- Submit feedback with curl to the feedback API.”
echo “- Use jq to view user comments from the feedback file.”
}
# Command to implement security measures
implementSecurityMeasures() {
echo “Function: Implement Security Measures”
echo “Actions:”
echo “- Use nmap for network scanning and security assessment.”
echo “- Use nikto for web server and CGI scanning.”
echo “- Review security-related commits in the git log.”
}
# Function for continuous improvement culture
continuousImprovementCulture() {
echo “Function: Continuous Improvement Culture”
echo “Actions:”
echo “- Add a task to continuously improve the platform.”
echo “- View the task list using taskwarrior.”
echo “- Mark a task as done using taskwarrior.”
}
# Menu
while true; do
echo “=== ShareMonero Menu ===”
echo “1. Define Project Goals”
echo “2. Assemble Team”
echo “3. Encourage Open Source”
echo “4. Implement Governance Model”
echo “5. Implement Emotional Features”
echo “6. Gather User Feedback”
echo “7. Implement Security Measures”
echo “8. Continuous Improvement Culture”
echo “0. Exit”
read -p “Enter your choice (0-8): ” choice
case $choice in
1) defineProjectGoals ;;
2) assembleTeam ;;
3) encourageOpenSource ;;
4) implementGovernanceModel ;;
5) implementEmotionalFeatures ;;
6) gatherUserFeedback ;;
7) implementSecurityMeasures ;;
8) continuousImprovementCulture ;;
0) echo “Exiting. Goodbye!”; exit ;;
*) echo “Invalid choice. Please enter a number between 0 and 8.” ;;
esac
echo
done
“`
Now, each menu option provides a brief explanation of the function and the associated actions. Users can choose an option based on a better understanding of what each function does. Adjust the descriptions as needed for your project’s specifics.
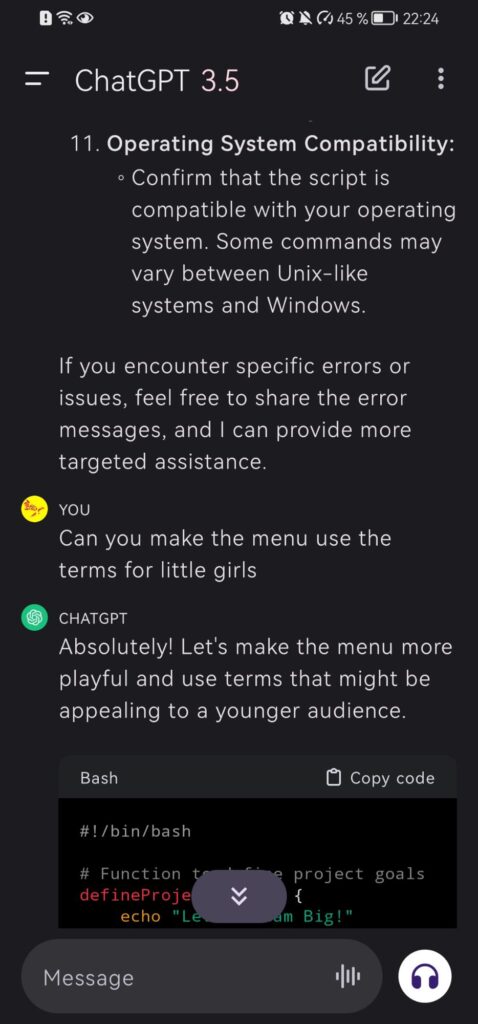
Absolutely! Let’s make the menu more playful and use terms that might be appealing to a younger audience.
“`bash
#!/bin/bash
# Function to define project goals
defineProjectGoals() {
echo “Let’s Dream Big!”
echo “Actions:”
echo “- Decide what we want to achieve with ShareMonero.”
echo “- Plan exciting milestones for our journey.”
echo “- Create a timeline to make our dreams come true.”
}
# Command to assemble the team
assembleTeam() {
echo “Calling All Super Friends!”
echo “Actions:”
echo “- Join our special team and get ready for adventures.”
echo “- Form a team by creating a magical branch.”
echo “- Add and commit changes to kick off our super team!”
}
# Command to encourage open source
encourageOpenSource() {
echo “Become a Code Wizard!”
echo “Actions:”
echo “- Create your own magical version of ShareMonero.”
echo “- Share your magical enhancements with everyone.”
echo “- Suggest exciting improvements to make ShareMonero even more magical!”
}
# Command to implement governance model
implementGovernanceModel() {
echo “Let’s Make Decisions Together!”
echo “Actions:”
echo “- Create a magical council (DAO) for decision-making.”
echo “- Install a voting spell to let everyone have a say.”
echo “- Cast your vote on enchanting proposals using the AragonCLI!”
}
# Command to implement emotional features
implementEmotionalFeatures() {
echo “Spread Happiness Spells!”
echo “Actions:”
echo “- Use magic to add happy features to our special place.”
echo “- Cast heartwarming spells to create a joyful atmosphere.”
echo “- Share enchanted images and make our space even more delightful!”
}
# Command to gather user feedback
gatherUserFeedback() {
echo “Let’s Hear Your Magical Thoughts!”
echo “Actions:”
echo “- Use a magical scroll (curl) to share your thoughts with us.”
echo “- Cast spells to send positive feedback and make our magic stronger.”
echo “- Check the enchanted scroll (feedback.json) to see what everyone is saying!”
}
# Command to implement security measures
implementSecurityMeasures() {
echo “Protect Our Magical Kingdom!”
echo “Actions:”
echo “- Use a magical map (nmap) to ensure our kingdom is secure.”
echo “- Cast spells (nikto) to check for any hidden dangers.”
echo “- Review the magic scroll (git log) for any signs of mischief.”
}
# Function for continuous improvement culture
continuousImprovementCulture() {
echo “Let’s Make Magic Even Better!”
echo “Actions:”
echo “- Add spells to continuously improve our magical kingdom.”
echo “- Use a magical list (taskwarrior) to keep track of our enchantments.”
echo “- Mark spells as done once we’ve made our magic stronger!”
}
# Menu
while true; do
echo “=== Enchanting ShareMonero Adventure ===”
echo “1. Dream Big – Define Project Goals”
echo “2. Super Team Assemble – Join our Magical Team”
echo “3. Code Wizardry – Encourage Open Source”
echo “4. Magical Decisions – Implement Governance”
echo “5. Spread Happiness – Implement Emotional Features”
echo “6. Share Your Thoughts – Gather User Feedback”
echo “7. Protect Our Kingdom – Implement Security Measures”
echo “8. Make Magic Better – Continuous Improvement”
echo “0. Exit the Enchanted Kingdom”
read -p “Enter your magical choice (0-8): ” choice
case $choice in
1) defineProjectGoals ;;
2) assembleTeam ;;
3) encourageOpenSource ;;
4) implementGovernanceModel ;;
5) implementEmotionalFeatures ;;
6) gatherUserFeedback ;;
7) implementSecurityMeasures ;;
8) continuousImprovementCulture ;;
0) echo “Exiting the Enchanted Kingdom. Farewell, little sorcerer!”; exit ;;
*) echo “Invalid choice. Please enter a number between 0 and 8.” ;;
esac
echo
done
“`
Feel free to customize the terms and descriptions further to create an engaging and playful experience for the intended audience.
Leave a Reply